CS 480/680 Class Projects
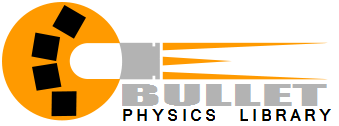
PA8 - Bullet
Objective:
- The purpose of this assignment is to become familiar with Bullet Physics, a 3D physics simulation engine. At the end of the assignment, you should be able to integrate 3D physics using Bullet into an OpenGL program with user interaction.
- Bullet Physics is a 3D multi-physics engine that provides essential functionality for a graphics application to seem real. It performs 3D collision detection, rigid body, and soft body dynamics. It provides many rudimentary shapes that you can use such as boxes, spheres, and cylinders along with triangle meshes.
- Bullet can be found at http://bulletphysics.org/wordpress/.
- It is recommended that you download bullet, Go through the documentation (the documentation is in the root of the source zip file), and examine the demos.
- Build a simple board (bottom with side walls) with 3 objects on it (a sphere, a cube, and a cylindar).
- Make the Cylindar a static object and the sphere and cube dynamic objects.
- provide input so that they can move ... in particular you want to be able to have the cube hit the ball and make it move...and then bounce off the walls and the cylindar.
- In Bullet, an object is dynamic if the mass and inertia are specified for it during its construction (look in Physics.cpp in functions addBall and addDynamicCylinder). These object's motion is controlled by rigid body dynamics (i.e. forces acting on them). You can interface with them through the btRigidBody and btMotionState classes.
- A static object in bullet is one which that has zero
mass and is fixed to a location. They do not move and are
just collision objects. These types of objects cannot
be animated either. But in many cases, we want objects
that we need to animate(not by applying a force on them)
and so that other objects (dynamic objects) to bounce
off of them. Bullet allows us to do this by making fixed
(or static) objects into kinematic objects. These types
of objects have physical properties such as friction
and restitution so that Bullet knows how dynamic objects
interact with them. There are two steps to make an object
kinematic :
//body is a btRigidBody pointer referencing the object being converted body->setCollisionFlags(body->getCollisionFlags() | btCollisionObject::CF_KINEMATIC_OBJECT); body->setActivationState(DISABLE_DEACTIVATION);
You can't apply a force on kinematic objects, like you did on the sphere, to move them. You have to translate them in the simulated world. - Notice how the kinematic cube collides with the dynamic object but it does not collide any with other static objects. This is because kinematic objects move like standalone bodies in a vacuum: there will be only one-way interaction: dynamic objects will be pushed away but there is no influence from dynamics objects
- Another issue with kinematic objects is that they apply a lot of force on dynamic objects(How would you resolve this issue?). Hint : Clamp the velocity?
- Suppose that you want to rotate the board so that the sphere rolls around it. To rotate the board, you have to convert it to a kinematic body and when you do rotate it, the board will exert a large force on the sphere. ( How would you solve this issue? [some research is required] ) Hint :What if you don't physically rotate the board, but project the angle of gravity onto the board(as if the board was rotated) and apply that force vector on the ball?
- Bullet allows you to build collision shapes using triangle meshes (using btTriangleMeshShape and btTriangleMesh objects). But primitive shapes have collision algorithms that are very fast and efficient and are suggested if possible. And Bullet assumes that you will handle all the collision for them (done by subclassing from Bullet's internal classes). "Primitives have specialist collision algorithms that are very fast. One should always attempt to use a primitive if it is the right shape."
- Load a triangle mesh into Bullet instead of using primitive objects.
- Assigned: Week 8
- Due: Week 10
- A clone of your GitHub repository will be made at 6:00am on
the due date. Don't forget to push your code by that time.
This clone will be evaluated and your grade posted.